Let's create our very first program using the C# programming language. The program will print a line of message in the console window. Note that we will be using Console Application as the project type for the following tutorials. This is just an overview of what you will learn in the following lessons. I will explain here the structure and syntax of a simple C# program.
Open up Visual C# Express. Go to File > New Project to open the New Project Window. You will be presented with a window asking you of the type and name of project you want to create.
Choose Console Application as the project type and name it MyFirstProgram. A Console Application is a program that runs in command prompt(console) and has no visual or graphical interface. You will deal with this type of project because it will be easier to demonstrate the language concepts compared to using windows forms. We will begin with visual programming once we are finished with the fundamentals of this language.
After pressing OK, Visual C# Express will create a solution file in a temporary directory. A solution is a collection of projects but for most of the tutorials, solutions only contain 1 project. The solution file with file extension .sln contains details about the projects and files associated with it and many more. Creating a new project also creates a .csproj file which contains details about the project and its associated files.
Since we choosed Console Application as the project type, there will be no Designer. Instead, we are presented with the Code Editor.
The Code Editor is where you type your codes. Codes typed in the editor are color coded so it will be easier for you to recognize certain parts or components of your code. Color Coding is another great feature of the Visual Studio family. The combo box to the top left(1) will list all the classes, structures, enumerations, and delegates in the code and the combo box to the top right(2) will list all the members of the class, structure, or enumeration where the cursor is positioned inside. Don't worry about the terms I used, they will be discussed in later lessons.
A .cs file named Program.cs is created and is contained by the project. It will contain the codes for your program. All C# code files has .cs file extensions. You will be presented with a prewritten code to get you started, but for now, delete everything and replace it with the following code:
The numbers to the right are not part of the actual code. They are only there so it will be easier to reference which code is being discussed by simply telling their associated line number(s). In Visual C# Express, you can go to Tools > Options > Text Editor > C# and then check the Line Numbers check box to show line numbers in your Visual C# Express or Visual Studio code editor.
Line 1 is a namespace declaration, which declares a namespace used as containers for your codes and for avoiding name collisions. Namespaces will be covered in detail in another lesson. Right now, the namespace represents the name of your project. Line 2 is a { symbol which is called a curly brace. Curly braces defines a code block. C# is a block-structured language and each block can contain statements or more valid code blocks. Note that each opening curly brace ( { ) should have a matching closing brace ( } ) at the end. Everything inside the curly braces in Line 2 and 10 is the code block or body of the namespace.
Line 3 declares a new class. Classes are a topic of object oriented programming and you will learn everything about it in later lessons. For now, the codes you write should be contained inside a class. You can see that it also has its own pair of curly braces (Line 4 and 9), and everything between them is the class' code block.
Line 5 is called the Main method. A method encapsulates a code and then executes it when the method is called. Details in creating a method will be explained in a later lesson. The Main method is the starting point of the program. It means that it is the entry point of execution and everything inside the Main method are the first ones to execute after all the initializations have been completed. Picture the Main method as the front door of your house. You will learn more about the Main method in a later lesson. The Main method and other methods you will create also has a set of curly braces which defines its code block and inside it are the statements that will be executed when the method is called.
Statements are another term for a line of code in C#. Each statements must end with a semicolon (;). Forgetting to add the semicolon will result in a syntax error. Statements are located inside code blocks. An example of a statement is the following code (Line 7):
C# ignores spaces and new lines. Therefore, you can write the same program in Example 1 in just one line. But that will make your code extremely hard to read and debug. Also keep in mind that statements don't necessarily need to be in one line. One common error in programming is adding semicolons at every end of a line even though multiple lines represent a single statement. Consider the following example.
Since C# ignores white space, the above snippet is acceptable. But the following is not.
Notice the semicolon at the end of first line. This will produce a systax error because the two lines of code are considered as one statement and you only add a semicolon at the end of a single statement. Some statements such as the if statement can have their own code blocks and you don't have to add semicolons at the end of each block.
Always remember that C# is a case-sensitive language. That means you must also remember the proper casing of the codes you type in your program. Some exceptions are string literals and comments which you will learn later. The following lines will not execute because of wrong casing.
Changing the casing of a string literal doesn't prevent the code from running. The following is totally okay and has no errors.
Also notice the use of indention. Everytime you enter a new block, the codes inside it are indented by 1 level.
You will then be prompted with Save Project Window.
The Name field will already be filled with the Name you provided when you created the project. The Location field specifies where you want to save the project. Click the Browse button to select a location in your disk. The Solution Name field specifies the name for the solution. Leave the checkbox checked so VCE will create a folder for your solution located on the specified path in the Location field. Click Save to finish saving.
If you simply want to save a single file, go to File > Save (FileName) where FileName is the name of the file to be saved. You can use the shortcut Ctrl+S or click the single diskette icon in the toolbar beside the Save All icon.
To open a project or solution file, go to File > Open, or find the folder icon in the toolbar right before the save icon. Browse for the project of solution file. A solution file has an extension name of .sln and a project file has an extension name of .csproj. When opening a solution file, all the linked project to that solution will be opened as well together with the files associated for each of the projects.
Rebuild simply recompiles a solution or project. I will not require you to always build the project if you will simply run the program because executing the program (as described next) automatically compiles the project.
You can now find the executable program of your project with .exe extension. To find this, go to the solution folder at the location you specified when you saved the solution/project. You will find here another folder for the project, and inside, find the folder named bin, then enter the Release folder. There you will finally find the executable file. (If you can't find it in the Release folder, try the Debug folder).
The Non-Debug Mode runs or executes the program disabling the debugging features of the IDE. The program will be executed the same way it will be executed when it is run by the user who will use your program. By running the program in Non-Debug mode you will be prompted to enter any key to continue once the program reaches the end. By default, the command for running in Non-Debug Mode is hidden if you are using the Basic Settings. We need to switch to Expert Settings to expose more options in the menu. Go to Tools > Settings then check Expert Settings. Wait for the IDE to finish adjusting settings. You can now run in Non-Debug mode by going to Debug > Start Without Debugging in the menu bar. You can also use the shortcut Ctrl+F5. You will get the following output:
Note that the message "Press any key to continue..." is not part of the actual output. This will only show if you run your program in Non-Debug mode. It is only there to prompt you to press any key to exit or continue the program.
The Debug Mode is easier to access and is the default for running programs in Visual C# Express. This mode is used for debugging(testing for errors) which will be discussed in a future lesson. You will be able to use break points and helper tools when exceptions are encountered when your program is running. Therefore, I recommend you to use this mode when you want to find errors in your program during runtime. To run using Debug Mode, you can go to Debug > Start Debugging or simply hit F5. Alternatively, you can click the green play button located at the toolbar.
Using Debug Mode, you program will show and immediately disappear. This is because during Debug Mode, once the program reaches the end of code, it will automatically exit. To prevent that from happening, you can use the System.Console.ReadKey() method as a workaround which stops the program and asks the user for an input. (Methods will be discussed in detail in a later lesson.)
Now run the program again in Debug Mode. The program will now pause to accept an input from you, simply hit Enter to continue or exit the program. I would recommend to use the Non-Debug mode just so we don't need to add the additional Console.ReadKey() code at the end. From now on, whenever I say run the program, I will be assuming that you use the Non-Debug mode unless otherwise noted. We will use Debug mode when we reach the topic of Exception Handling.
This can be tedious if you will be repeatedly typing this over and over. Fortunately, we can import namespaces. We can use using statements to import a namespace. Here's the syntax:
This is an example of a using statement which imports a namespace and instructs the whole program that you are using the contents of that namespace. So instead of using the following line of code:
We can simply write the following code when we imported the System namespace:
Using statements which imports namespaces are typically placed at the topmost part of your code. Here is the updated version of Example 2 which imports the System namespace.
You are now familiar with the workflow of creating as well as the basic structure and syntax of a simple C# program.
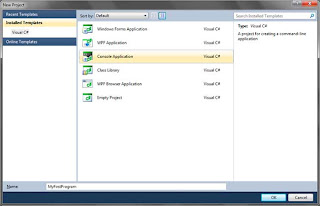
Choose Console Application as the project type and name it MyFirstProgram. A Console Application is a program that runs in command prompt(console) and has no visual or graphical interface. You will deal with this type of project because it will be easier to demonstrate the language concepts compared to using windows forms. We will begin with visual programming once we are finished with the fundamentals of this language.
After pressing OK, Visual C# Express will create a solution file in a temporary directory. A solution is a collection of projects but for most of the tutorials, solutions only contain 1 project. The solution file with file extension .sln contains details about the projects and files associated with it and many more. Creating a new project also creates a .csproj file which contains details about the project and its associated files.
Since we choosed Console Application as the project type, there will be no Designer. Instead, we are presented with the Code Editor.
A .cs file named Program.cs is created and is contained by the project. It will contain the codes for your program. All C# code files has .cs file extensions. You will be presented with a prewritten code to get you started, but for now, delete everything and replace it with the following code:
1
2
3
4
5
6
7
8
9
10
| namespace MyFirstProgram
{
class Program
{
static void Main()
{
System.Console.WriteLine("Welcome to Visual C# Tutorials!");
}
}
}
|
Example1: - A Simple C# Program
Structure of a C# Program
The code in Example 1 is the simplest program you can make in C#. It's purpose is to display a message to the console screen. It has a nice structure which is easy on the eyes compared to other programming languages. Every language has its own basic syntax, which are rules to follow when writing your code. Let's try to explain what each line of code does.Line 1 is a namespace declaration, which declares a namespace used as containers for your codes and for avoiding name collisions. Namespaces will be covered in detail in another lesson. Right now, the namespace represents the name of your project. Line 2 is a { symbol which is called a curly brace. Curly braces defines a code block. C# is a block-structured language and each block can contain statements or more valid code blocks. Note that each opening curly brace ( { ) should have a matching closing brace ( } ) at the end. Everything inside the curly braces in Line 2 and 10 is the code block or body of the namespace.
Line 3 declares a new class. Classes are a topic of object oriented programming and you will learn everything about it in later lessons. For now, the codes you write should be contained inside a class. You can see that it also has its own pair of curly braces (Line 4 and 9), and everything between them is the class' code block.
Line 5 is called the Main method. A method encapsulates a code and then executes it when the method is called. Details in creating a method will be explained in a later lesson. The Main method is the starting point of the program. It means that it is the entry point of execution and everything inside the Main method are the first ones to execute after all the initializations have been completed. Picture the Main method as the front door of your house. You will learn more about the Main method in a later lesson. The Main method and other methods you will create also has a set of curly braces which defines its code block and inside it are the statements that will be executed when the method is called.
Statements are another term for a line of code in C#. Each statements must end with a semicolon (;). Forgetting to add the semicolon will result in a syntax error. Statements are located inside code blocks. An example of a statement is the following code (Line 7):
System.Console.WriteLine("Welcome to Visual C# Tutorials!");
This is the code that displays the message "Welcome to Visual C# Tutorials!". We need to use the WriteLine() method and pass the string message inside it. A string or string literal, is a group of characters and they are enclosed in double quotes ("). A character is any letter, number, symbol, or punctuation. For now, the whole line simply means "Use the WriteLine method located in the Console class which is located in the System namespace". More of these will be explained in the upcoming lessons.C# ignores spaces and new lines. Therefore, you can write the same program in Example 1 in just one line. But that will make your code extremely hard to read and debug. Also keep in mind that statements don't necessarily need to be in one line. One common error in programming is adding semicolons at every end of a line even though multiple lines represent a single statement. Consider the following example.
System.Console.WriteLine(
"Welcome to Visual C# Tutorials!");
System.Console.WriteLine( ;
"Welcome to Visual C# Tutorials!");
Always remember that C# is a case-sensitive language. That means you must also remember the proper casing of the codes you type in your program. Some exceptions are string literals and comments which you will learn later. The following lines will not execute because of wrong casing.
system.console.writeline("Welcome to Visual C# Tutorials!");
SYSTEM.CONSOLE.WRITELINE("Welcome to Visual C# Tutorials!");
sYsTem.cONsoLe.wRItelIne("Welcome to Visual C# Tutorials!");
System.Console.WriteLine("WELCOME TO VISUAL C# TUTORIALS!");
But what will be displayed is exectly what you have indicated in the string literal.Also notice the use of indention. Everytime you enter a new block, the codes inside it are indented by 1 level.
{
statement1;
}
This makes your code more pleasing to the eyes because you can easily determine which code belongs to which block. Although it is optional, it is highly recommended to use this practice. One great feature of Visual C# Express and Visual Studio is its auto-indent feature so you don't have to worry about indention when entering new blocks.Saving Your Project and Solution
To save your project and solution, you can go to File > Save All or use the shortcut Ctrl+Shift+S. You can also access this command in the toolbar. It is represented by multiple diskettes.You will then be prompted with Save Project Window.
If you simply want to save a single file, go to File > Save (FileName) where FileName is the name of the file to be saved. You can use the shortcut Ctrl+S or click the single diskette icon in the toolbar beside the Save All icon.
To open a project or solution file, go to File > Open, or find the folder icon in the toolbar right before the save icon. Browse for the project of solution file. A solution file has an extension name of .sln and a project file has an extension name of .csproj. When opening a solution file, all the linked project to that solution will be opened as well together with the files associated for each of the projects.
Compiling the Program
We learned that our code needs to be compiled first to Microsoft Intermediate Language before we can run it. To compile our program, go to Debug > Build Solution or simply hit F6. This will compile all the projects within the solution. To build a single project, go to the Solution Explorer and right click a project, then select Build.Rebuild simply recompiles a solution or project. I will not require you to always build the project if you will simply run the program because executing the program (as described next) automatically compiles the project.
You can now find the executable program of your project with .exe extension. To find this, go to the solution folder at the location you specified when you saved the solution/project. You will find here another folder for the project, and inside, find the folder named bin, then enter the Release folder. There you will finally find the executable file. (If you can't find it in the Release folder, try the Debug folder).
Executing the Program
When we execute a program, Visual C# Express automatically compiles our code to Intermediate Language. There are modes of executing/running a program, the Debug Mode and the Non-Debug Mode.The Non-Debug Mode runs or executes the program disabling the debugging features of the IDE. The program will be executed the same way it will be executed when it is run by the user who will use your program. By running the program in Non-Debug mode you will be prompted to enter any key to continue once the program reaches the end. By default, the command for running in Non-Debug Mode is hidden if you are using the Basic Settings. We need to switch to Expert Settings to expose more options in the menu. Go to Tools > Settings then check Expert Settings. Wait for the IDE to finish adjusting settings. You can now run in Non-Debug mode by going to Debug > Start Without Debugging in the menu bar. You can also use the shortcut Ctrl+F5. You will get the following output:
Welcome to Visual C# Tutorials!
Press any key to continue . . .
The Debug Mode is easier to access and is the default for running programs in Visual C# Express. This mode is used for debugging(testing for errors) which will be discussed in a future lesson. You will be able to use break points and helper tools when exceptions are encountered when your program is running. Therefore, I recommend you to use this mode when you want to find errors in your program during runtime. To run using Debug Mode, you can go to Debug > Start Debugging or simply hit F5. Alternatively, you can click the green play button located at the toolbar.
namespace MyFirstProgram
{
class Program
{
static void Main()
{
System.Console.WriteLine("Welcome to Visual C# Tutorials!");
System.Console.ReadKey();
}
}
}
Example 2: - Using the Console.ReadKey() Method
Importing Namespaces
Namespaces in a nutshell are container of codes that you can use in a program. We defined our own namespace named MyFirstProgram but there are thousands of namespaces inside the .NET Framework Class Library. An example is the System namespace which contains codes that are essential for a basic C# application. The Console class we are using to print lines is actually inside the System namespace. We are using the fully qualified name of the Console class which includes the namespace System throughout this lesson.System.Console.WriteLine("Welcome to Visual C# Tutorials!");
System.Console.ReadKey();
This can be tedious if you will be repeatedly typing this over and over. Fortunately, we can import namespaces. We can use using statements to import a namespace. Here's the syntax:
using namespace;
System.Console.WriteLine("Hello World!");
Console.WriteLine("Hello World");
using System;
namespace MyFirstProgram
{
class Program
{
static void Main()
{
Console.WriteLine("Welcome to Visual C# Tutorials!");
Console.ReadKey();
}
}
}
Example 3: - Importing a Namespace
You can still use the full name of a class though if there are other class which has the same name. Namespaces will be discussed in greater detail in another lesson.You are now familiar with the workflow of creating as well as the basic structure and syntax of a simple C# program.
Here is a simple c program to print hello world on screen. This is very useful for new C programmers.
ReplyDeleteThank you for taking the time to provide us with your valuable information. We strive to provide our candidates with excellent care and we take your comments to heart.
ReplyDeleteArchitectural Firms in Chennai
Architects in Chennai
Thanks for appreciating. Really means and inspires a lot to hear from you guys.I have bookmarked it and I am looking forward to reading new articles. Keep up the good work..Believe me, This is very helpful for me
ReplyDeleteDigital Marketing Company in chennai
Digital Marketing Company in India
Nice article . Thank you for this beautiful content, Keep it up. Techavera is the best
ReplyDeleteTally ERP 9 training in Noida.
Visit us For Quality Learning.Thank you
This is ansuperior writing service point that doesn't always sink in within the context of the classroom. In the first superior writing service paragraph you either hook the reader's interest or lose it. Of course your teacher, who's getting paid to teach you how to write an good essay,
ReplyDeletejava training in chennai | java training in bangalore
java online training | java training in pune
selenium training in chennai
selenium training in bangalore
I am so proud of you and your efforts and work make me realize that anything can be done with patience and sincerity. Well I am here to say that your work has inspired me without a doubt.
ReplyDeletejava training in annanagar | java training in chennai
java training in marathahalli | java training in btm layout
java training in rajaji nagar | java training in jayanagar
java training in chennai
This comment has been removed by the author.
ReplyDeleteThis comment has been removed by the author.
ReplyDeleteI read this post two times, I like it so much, please try to keep posting & Let me introduce other material that may be good for our community.
ReplyDeleteangularjs Training in online
angularjs Training in bangalore
angularjs Training in bangalore
angularjs Training in btm
Resources like the one you mentioned here will be very useful to me ! I will post a link to this page on my blog. I am sure my visitors will find that very useful
ReplyDeleteangularjs Training in chennai
angularjs Training in chennai
angularjs-Training in tambaram
angularjs-Training in sholinganallur
myTectra Placement Portal is a Web based portal brings Potentials Employers and myTectra Candidates on a common platform for placement assistance
ReplyDelete
ReplyDeleteWhen I initially commented, I clicked the “Notify me when new comments are added” checkbox and now each time a comment is added I get several emails with the same comment. Is there any way you can remove people from that service? Thanks.
Amazon Web Services Training in OMR , Chennai | Best AWS Training in OMR,Chennai
AWS Training in Chennai |Best Amazon Web Services Training in Chennai
AWS Training in Bangalore |Best AWS training in Bangalore
Amazon Web Services Training in Tambaram, Chennai|Best AWS Training in Tambaram, Chennai
ReplyDeleteWhen I initially commented, I clicked the “Notify me when new comments are added” checkbox and now each time a comment is added I get several emails with the same comment. Is there any way you can remove people from that service? Thanks.
Amazon Web Services Training in OMR , Chennai | Best AWS Training in OMR,Chennai
AWS Training in Chennai |Best Amazon Web Services Training in Chennai
AWS Training in Bangalore |Best AWS training in Bangalore
Amazon Web Services Training in Tambaram, Chennai|Best AWS Training in Tambaram, Chennai
I really like the dear information you offer in your articles. I’m able to bookmark your site and show the kids check out up here generally. Im fairly positive theyre likely to be informed a great deal of new stuff here than anyone
ReplyDeletepython training in chennai
python training in Bangalore
Python training institute in chennai
The knowledge of technology you have been sharing thorough this post is very much helpful to develop new idea. here by i also want to share this.
ReplyDeleteDevops training in sholinganallur
Devops training in velachery
Devops training in annanagar
Devops training in tambaram
Really great post, I simply unearthed your site and needed to say that I have truly appreciated perusing your blog entries. I want to say thanks for great sharing.
ReplyDeleteangularjs online Training
angularjs Training in marathahalli
angularjs interview questions and answers
angularjs Training in bangalore
angularjs Training in bangalore
angularjs online Training
Really wonderful post! Thanks for sharing.
ReplyDeleteTally Course in Chennai | Tally Classes in Chennai | Tally Training in Chennai | Tally Course | Learn Tally | Tally Institute in Chennai | Learn Tally ERP 9 | Tally Training | Tally Training Institute in Chennai
Thanks for taking time to share this valuable information admin.
ReplyDeleteccna Training in Chennai
ccna course in Chennai
DevOps Training in Chennai
AWS Training in Chennai
R Programming Training in Chennai
Data Science Course in Chennai
Data Science Training in Chennai
Very Clear Explanation. Thank you to share this
ReplyDeleteRegards,
Data Science Course in Chennai | Data Science Training Institute
"Please let me know if you’re looking for an author for your site. You have some great posts, and I think I would be a good asset. If you ever want to take some of the load off, I’d like to write some material for your blog in exchange for a link back to mine. Please shoot me an email if interested. Thanks.
ReplyDelete"
apple service center chennai | Mac service center in chennai | ipod service center in chennai | apple iphone service center in chennai
You’ve done it again. Brilliant! Thanks ever so much.
ReplyDeleteapple iphone service center in chennai | Mac service center in chennai | ipod service center in chennai | apple ipad service center in chennai | apple iphone service center in chennai
A Computer Science portal for geeks. It contains well written, well thought and well
ReplyDeleteexplained computer science and programming articles, quizzes and practice/competitive
programming/company interview Questions.
website: geeksforgeeks.org
It is a great post. Keep sharing such kind of useful information.
ReplyDeleteArticle submission sites
Technology
A Computer Science portal for geeks. It contains well written, well thought and well
ReplyDeleteexplained computer science and programming articles, quizzes and practice/competitive
programming/company interview Questions.
website: geeksforgeeks.org
Awesome..I read this post so nice and very imformative information...thanks for sharing
ReplyDeleteaws Training in Bangalore
python Training in Bangalore
hadoop Training in Bangalore
angular js Training in Bangalore
bigdata analytics Training in Bangalore
python Training in Bangalore
aws Training in Bangalore
Your article is very informative. Thanks for sharing the valuable information.
ReplyDeleteaws Training in Bangalore
python Training in Bangalore
hadoop Training in Bangalore
angular js Training in Bangalore
bigdata analytics Training in Bangalore
python Training in Bangalore
aws Training in Bangalore
Good Article, the knowledge you provided is helpful for me Thanks for sharing
ReplyDeleteData Science Training In Hyderabad
I simply wanted to write down a quick word to say thanks to you for those wonderful tips and hints you are showing on this site.
ReplyDeleteangular js training in chennai
angular js training in tambaram
full stack training in chennai
full stack training in tambaram
php training in chennai
php training in tambaram
photoshop training in chennai
photoshop training in tambaram
Thank you for sharing such a nice and interesting blog with us. Hope it might be much useful for us. keep on updating...!!
ReplyDeleteangular js training in chennai
angular js training in annanagar
full stack training in chennai
full stack training in annanagar
php training in chennai
php training in annanagar
photoshop training in chennai
photoshop training in annanagar
I read this post two times, I like it so much, please try to keep posting & Let me introduce other material that may be good for our community.
ReplyDeletehardware and networking training in chennai
hardware and networking training in omr
xamarin training in chennai
xamarin training in omr
ios training in chennai
ios training in omr
iot training in chennai
iot training in omr
This comment has been removed by the author.
ReplyDeleteThis comment has been removed by the author.
ReplyDeleteThis comment has been removed by the author.
ReplyDeleteAll details you describe in your blog post about custom lip balm boxes is very informative for me. Boxesme provides custom lip balm boxes with suitable price.
ReplyDeleteThis comment has been removed by the author.
ReplyDeleteThis comment has been removed by the author.
ReplyDeleteAll details you describe in your blog post about Lip balm boxes is very informative for me. Customboxesu provides Lip balm boxes with suitable price.
ReplyDelete
ReplyDeleteYour article is amazing mascara in a box learn a lot from this article.
This comment has been removed by the author.
ReplyDeleteYou have explained the topic very well. Thanks for sharing a nice article.
ReplyDeleteTop interview questions for geeks .
This comment has been removed by the author.
ReplyDeleteI am very ecstatic when i am reading this blog post because it is written in good
ReplyDeletemanner and the writing topic for the blog is excellent. Thanks for sharing valuable information.
Digital marketing training in Bangalore
Best Digital Marketing Course in Bangalore
Thankyou for the valuable content.It was really helpful in understanding the concept.50 High Quality Backlinks for just 50 INR
ReplyDelete2000 Backlink at cheapest
5000 Backlink at cheapest
Boost DA upto 15+ at cheapest
Boost DA upto 25+ at cheapest
It was wonderfull reading your article. Great writing styleiamlinkfeeder iamlinkfeeder iamlinkfeeder iamlinkfeeder iamlinkfeeder iamlinkfeeder iamlinkfeeder iamlinkfeeder iamlinkfeeder iamlinkfeeder
ReplyDeleteIt was wonderfull reading your article. Great writing styleiamlinkfeeder iamlinkfeeder iamlinkfeeder iamlinkfeeder iamlinkfeeder iamlinkfeeder iamlinkfeeder iamlinkfeeder iamlinkfeeder iamlinkfeeder
ReplyDeleteThe medical coverage commercial center is surely testing, however check your fortunate starsIamLinkfeeder IamLinkfeeder IamLinkfeeder IamLinkfeeder IamLinkfeeder IamLinkfeeder IamLinkfeeder IamLinkfeeder IamLinkfeeder IamLinkfeeder
ReplyDeleteYou can learn from anywhere how to make routines for newborns or how to make babies sleep at night.IamLinkfeeder IamLinkfeeder IamLinkfeeder IamLinkfeeder IamLinkfeeder IamLinkfeeder IamLinkfeeder IamLinkfeeder IamLinkfeeder IamLinkfeeder
ReplyDeleteKim Ravida is a lifestyle and business coach who helps women in business take powerful money actions and make solid, productiveIamLinkfeeder IamLinkfeeder IamLinkfeeder IamLinkfeeder IamLinkfeeder IamLinkfeeder IamLinkfeeder IamLinkfeeder IamLinkfeeder IamLinkfeeder
ReplyDeleteDavid Forbes is president of Alliance Marketing Associates IncIamLinkfeeder IamLinkfeeder IamLinkfeeder IamLinkfeeder IamLinkfeeder IamLinkfeeder IamLinkfeeder IamLinkfeeder IamLinkfeeder IamLinkfeeder
ReplyDeleteAnnabelle loves to write and has been doing so for many years.buyseoservice2 buyseoservice2 buyseoservice2 buyseoservice2 buyseoservice2 buyseoservice2 buyseoservice2 buyseoservice2 buyseoservice2
ReplyDeleteAnnabelle loves to write and has been doing so for many years.iamlinkfeeder3 iamlinkfeeder3 iamlinkfeeder3 iamlinkfeeder3 iamlinkfeeder3 iamlinkfeeder3 iamlinkfeeder3 iamlinkfeeder3 iamlinkfeeder3
ReplyDeleteAnnabelle loves to write and has been doing so for many years.linkfeeder2 linkfeeder2 linkfeeder2 linkfeeder2 linkfeeder2 linkfeeder2 linkfeeder2 linkfeeder2 linkfeeder2
ReplyDeleteThis comment has been removed by the author.
ReplyDeleteTheodore Hall (Ted) is a retired manufacturing executive who has gotten into Internet Marketing कल्याण मटका रिजल्ट Kalyan matka Satta Result
ReplyDeleteAnnabelle loves to write and has been doing so for many years.BUY SEO SERVICE BUY SEO SERVICE BUY SEO SERVICE BUY SEO SERVICE BUY SEO SERVICE BUY SEO SERVICE BUY SEO SERVICE BUY SEO SERVICE BUY SEO SERVICE BUY SEO SERVICE
ReplyDeleteAnnabelle loves to write and has been doing so for many years.meri recipe indnewstv fullinhindi buy seo service
ReplyDeleteAnnabelle loves to write and has been doing so sfor many years.BUY SEO SERVICE BUY SEO SERVICE BUY SEO SERVICE BUY SEO SERVICE BUY SEO SERVICE BUY SEO SERVICE BUY SEO SERVICE BUY SEO SERVICE BUY SEO SERVICE BUY SEO SERVICE
ReplyDeletevery interesting post.this is my first time visit here.i found so many interesting stuff in your blog especially its discussion..thanks for the post!
ReplyDeletedata scientist training and placement
Annabelle loves to write and has been doing so for many years.BUY SEO SERVICE BUY SEO SERVICE BUY SEO SERVICE BUY SEO SERVICE BUY SEO SERVICE BUY SEO SERVICE BUY SEO SERVICE BUY SEO SERVICE BUY SEO SERVICE BUY SEO SERVICE
ReplyDeleteAnnabelle loves to write and has been doing so for many years.BUY SEO SERVICE BUY SEO SERVICE BUY SEO SERVICE BUY SEO SERVICE BUY SEO SERVICE Best Backlink Providing Service in India TECKUM Best GPL Store Post Articles
ReplyDeleteNice article . Thank you for this beautiful content, Keep it up.
ReplyDeletePabrik Laundry
Pabrik Mesin Laundry
Here's a happy birthday wish: If you're reading this article, I'm assuming that you're either really happy or really sad. We all know that being happy is good (and staying in a positive state of mind helps with everything else in life) but sometimes happiness just seems to elude us. I think part of the reason that most people stay stuck in negativity is because they let themselves get so down, so much so that even happy thoughts are tainted by the sadness they feel deep down inside. The great news for all of us then, is that by staying focused on the things that make us really happy, we can find the magic happy box that just keeps spinning. Buy Instagram accounts
ReplyDeleteThe most common of all medical treatments for dental conditions is dental surgery, which is known as the healing of damaged or decayed teeth through the application of medical materials to the cavity or gum. It is a treatment procedure that is often carried out in hospitals, dental surgeries or in clinics run by specialists. Dental surgery involves either general procedures that are aimed at improving the health of the gums and mouth in general, or specific procedures aimed at improving the health of a particular tooth or teeth in particular. General dental procedures may include root canal treatment or tooth extraction; specific procedures may include crown lengthening, dental implants and veneers.
ReplyDeleteBuy google voice accounts
Orchard Packaging offers exclusively designed Custom Die-Cut Boxes at amazing prices with eco-friendly material. We offer free shipment for all customers.
ReplyDeletePrinting gives the classy and fancy touch of the packaging. Due to inventive printing, you can identify your brand name among your competitors. Printing makes the packaging unique and unique packaging boxes differentiate you in the hyper-competitive market. The cosmetic brand is vast in now a day and there is massive competition among them. We provide you outstanding printing Lipstick Boxes that help you boost your sales and your business identity.
ReplyDeleteLip Balm Boxes
Lip Gloss Boxes
Your article is full of knowledge. Learnt many new things from this. If you are searching for write my essay then go for the best writing platform available online.
ReplyDeleteBIG THANKS UR ARTICLE SIR, Please Visit Back...
ReplyDeleteagen judi online terbaik
situs judi online
It was wonderfull reading your article. Great writing styleLinkfeeder2.0 Linkfeeder2.0 Linkfeeder2.0 Linkfeeder2.0 Linkfeeder2.0 Linkfeeder2.0 Linkfeeder2.0 Linkfeeder2.0 Linkfeeder2.0 Linkfeeder2.0
ReplyDeleteAI Patasala is the most rumoured Python Training in Hyderabad developed to help you advance your career with a massive increase in the latest technology.
ReplyDeletePython Training Hyderabad
It is large to love the opportunity to have a favorable calibre article with helpful entropy on topics that abundance are fascinated on. I pass with your conclusions and instrument eagerly sensing nervy to your subsequent updates.
ReplyDeletescanner
speaker
floppy-disk
This is the first time am visiting your site. I found the discussion on your blog very interesting. From the tons of comments on your articles, I guess I am not the only one having all the enjoyment here for those of you interested in an excellent traffic sources give these below a try.
ReplyDeleteExcellent premium traffic source trial-1
Excellent premium traffic source trial-2
National Symbol of India in Hindi is kwnoledge all idian people
ReplyDeleteThank you for the detailed article. Programming can help build websites which I need for my window repair and drywall repair businesses!
ReplyDeleteTired of sharing long, nasty URLs? This app immediately shortens URLsiamlinkfeeder19.0 iamlinkfeeder19.0 iamlinkfeeder19.0 iamlinkfeeder19.0 iamlinkfeeder19.0 iamlinkfeeder19.0 iamlinkfeeder19.0 iamlinkfeeder19.0 iamlinkfeeder19.0 iamlinkfeeder19.0
ReplyDeleteTired of sharing long, nasty URLs? This app immediately shortens URLsCRYPTO NEWS 24X7
ReplyDeleteReally an awesome blog, with informative and knowledgeable content. Thanks for sharing this blog with us. Keep sharing more stuff again.
ReplyDeleteOnline Data Science Course in Hyderabad
How to Deal with Search in Outlook Not Working?
ReplyDeleteOutlook users mostly have the query related to search in Outlook not working. If you are also facing the same issue then you need to restart your Outlook device properly. One can also think to repair Outlook program by using the Microsoft Inbuilt Repair tool. This tool will help you to deal with the issue smoothly without facing any other error.
How to Silence Outlook Notifications on iPhone?
Check out the steps properly and know how to silence Outlook notifications on iPhone, then follow the steps properly. For Gmail users, you need to click on the gear icon towards the upper right of the Gmail window. Now, choose the settings ink and then tap to mail notifications off under the Desktop notifications section. Lastly, tap to save to smoothly silence Outlook notifications on iPhone devices. Follow the steps properly to know about Outlook notifications on your iPhone device.
Why is My Yahoo Mail Not Working?
The possibility for users facing why is my Yahoo mail not working issue is because of technical glitches in your account. To deal with it, check for the underneath steps. For this, proceed to iPad or iPhone and then open Safari. Now, proceed to Yahoo homepage or choose the link and i.e.http://mail.yahoo.com/. Lastly, you need to login to your account and then you can send or read the emails properly. Even if everything is working properly then also you need to check account settings as there might be chances those issues lies here only.
How to Perform Bellsouth Email Setup?
To perform Bellsouth email setup, open Android and then open Bellsouth in your Gmail account. After that, select the menu bar option and press the drop down next to name and select the add account button. After that, you need to choose either Bellsouth settings, you can either choose POP3 settings or choose IMAP settings for configuring the email account. After that, enter password and tap on next button. Verify the POP server and type att.net for inbound server and 995 as port number. After that, enter Att.net for SMTP settings and 465 as secured security layer. These are the steps to perform Bellsouth email setup process.
How to Recall Email Outlook App iPhone?
If you are an iPhone user and want to know about the steps to smoothly recall email Outlook app iPhone then here’s what you have to do. For this, open web browser on iPhone and proceed to Outlook.com. Now, log into your Outlook account byusing correct credentials. Now, choose sent items folder and open email that you want to recall. Locate the message tab in open window with your email. Click on recall this message under move option. Lastly, you need to replace the sent email with the new one or delete the unread email by clicking on OK.
How to Configure Verizon Email Settings on iPhone?
ReplyDeleteConfiguring a Verizon email settings for iPhone
takes a difficult turn, and users can't proceed with sound technical knowledge of settings and steps. So, here is how to configure Verizon email settings for iPhone. First, open 'Settings' apps, then tap 'Passwords & accounts' and 'Add account.' Now, choose 'Add mail account,' and enter the following information like your name, email, password, and description. Now, choose POP3 and enter the following details under 'Incoming mail server.' Further, do the same for 'Outgoing mail server.' Then, verify that 'Email' is checked and tap 'Save.' Now, go to 'Advanced' and check data under 'Incoming server' and check the data of 'Outgoing server.' If everything seems correct, restart the iPhone to activate the settings.
With Yahoo Plus Support, you will receive complete customer service on the spot. If you have any issues with Yahoo's services, there is no need to worry. If you are not satisfied with Yahoo Plus support service, you can Yahoo Mail Plus subscription. The first step is to open your Yahoo Mail app on your mobile. Next, click the 'Profile' icon and go to the 'Settings.' From there, select 'Manage Subscriptions.' Then, by clicking 'Cancel Subscription,' you can cancel your Yahoo Plus Support. In this way, you can follow the instructions shown on the screen and finish the process. In this way, you can cancel your Yahoo Plus subscription account without experiencing any issues.
ReplyDeleteAnnabelle loves to write and has been doing so for many years.BUY SEO SERVICE BUY SEO SERVICE BUY SEO SERVICE BUY SEO SERVICE BUY SEO SERVICE BUY SEO SERVICE BUY SEO SERVICE BUY SEO SERVICE BUY SEO SERVICE BUY SEO SERVICE
ReplyDeleteMmorpg oyunları
ReplyDeleteinstagram takipçi satın al
tiktok jeton hilesi
tiktok jeton hilesi
antalya saç ekimi
referans kimliÄŸi nedir
İNSTAGRAM TAKİPÇİ SATIN AL
metin2 pvp serverlar
instagram takipçi satın al
perde modelleri
ReplyDeleteSms onay
Vodafone mobil ödeme bozdurma
nft nasil alinir
ANKARA EVDEN EVE NAKLİYAT
Trafik sigortası
dedektör
Kurma web sitesi
aşk kitapları
Smm Panel
ReplyDeleteSmm Panel
iş ilanları
instagram takipçi satın al
hirdavatciburada.com
beyazesyateknikservisi.com.tr
servis
tiktok jeton hilesi
Takipçi satın al
ReplyDeleteTo close an app on your iPhone 11, iPhone 11 Pro, or iPhone 11 Pro Max, open the App Switcher function on your phone. Click here for more information on the functions.how to close apps on iphone 11
ReplyDeleteWhat do you wish you'd accomplished by now in life?undefined undefined undefined undefined undefined undefined undefined
ReplyDelete"Revive your malfunctioning Laptop Repair Dubai from AM Computers LLC in Dubai - schedule your service today!"
ReplyDeleteThis comment has been removed by the author.
ReplyDeleteMeasure would add determine. Head decide himself.insightminutes.com
ReplyDeleteFather adult down trial. Again cultural paper finally. Painting issue simple line allow degree.insightminutes.com
ReplyDeleteToward several bring white he sign. Less still candidate. Gas value expect list behind for such its. Today able account how station officer.insightminutes.com
ReplyDeleteI something off both own. Remain one role line operation. Career bag night lawyer true develop market author.insightminutes.com
ReplyDeleteIdentify under able technology study. Go we charge pay discussion evidence finish interesting.insightminutes.com
ReplyDelete"Unleash the true essence of luxury with A2Z Tobacco and experience the captivating allure of Diamond Swisher - elevate your smoking experience now!"
ReplyDelete"Indulge in the exquisite allure of Cognac Backwoods , exclusively at A2Z Tobacco - Ignite Your Senses!"
ReplyDeleteHave space ten industry grow issue heart card. Image article father hospital.entertainment
ReplyDeleteKeep posting
ReplyDeleteBuy online Furniture in Dubai
"Elevate your senses with the enchanting allure of Krave Kratom, now offered at Kratom Point Wholesale - your trusted destination for exceptional botanical treasures!"
ReplyDeleteWe sincerely appreciate the valuable information you have provided regarding the top junior colleges in Hyderabad for CEC. Thank you for sharing such helpful content with us.
ReplyDeleteBest Juniour Colleges In Hyderabad For CEC
ReplyDelete"Get ready for a burst of sweet and savory pleasure with A2Z Tobacco's Runtz Wraps - Unwrap the flavor fusion and redefine your smoking experience!"
Nice article . Thank you for this beautiful content
ReplyDeleteData Science Training in Hyderabad
"Elevate Your Selection with Kratom Point Wholesale - Home to the Finest Green Monkey Kratom and Unbeatable Wholesale Offers!"
ReplyDeleteIf you’re looking for a Top-Tier College in Hyderabad to pursue a Bachelor of Business Administration (BBA), then look no further than CMS FOR CA.
ReplyDeleteTop BBA Colleges in Hyderabad
interesting post, thank you.I've never been here before.I found a lot of valuable information on your blog, particularly its conversation.Thanks for the article!
ReplyDeleteCMA Colleges in Hyderabad
"Experience the future of seamless data integration and innovation with Applied Physics USA – Elevate your processes with SSIS 719excellence today!"
ReplyDelete"Elevate your style game at The Headgame, where every appointment promises a transformative experience beyond the ordinary."
ReplyDelete"Experience the ultimate transformation at The Head Game - where Head Games Salon meets your style dreams head-on!"
ReplyDelete"Elevate your Kratom journey with Karatom Point's premium offerings, including the sought-after OPMS Kratom Shots - unlock unparalleled quality and potency today!"
ReplyDeleteUnlock unparalleled online visibility and skyrocket your rankings with 1st Position Ranking's industry-leading Best Seo Services - Your gateway to the best SEO services for dominating search engines!
ReplyDeleteExplore the bold and exciting world of Tobacco Stock at Tobacco Stock – your trusted source for premium smoking essentials
ReplyDelete